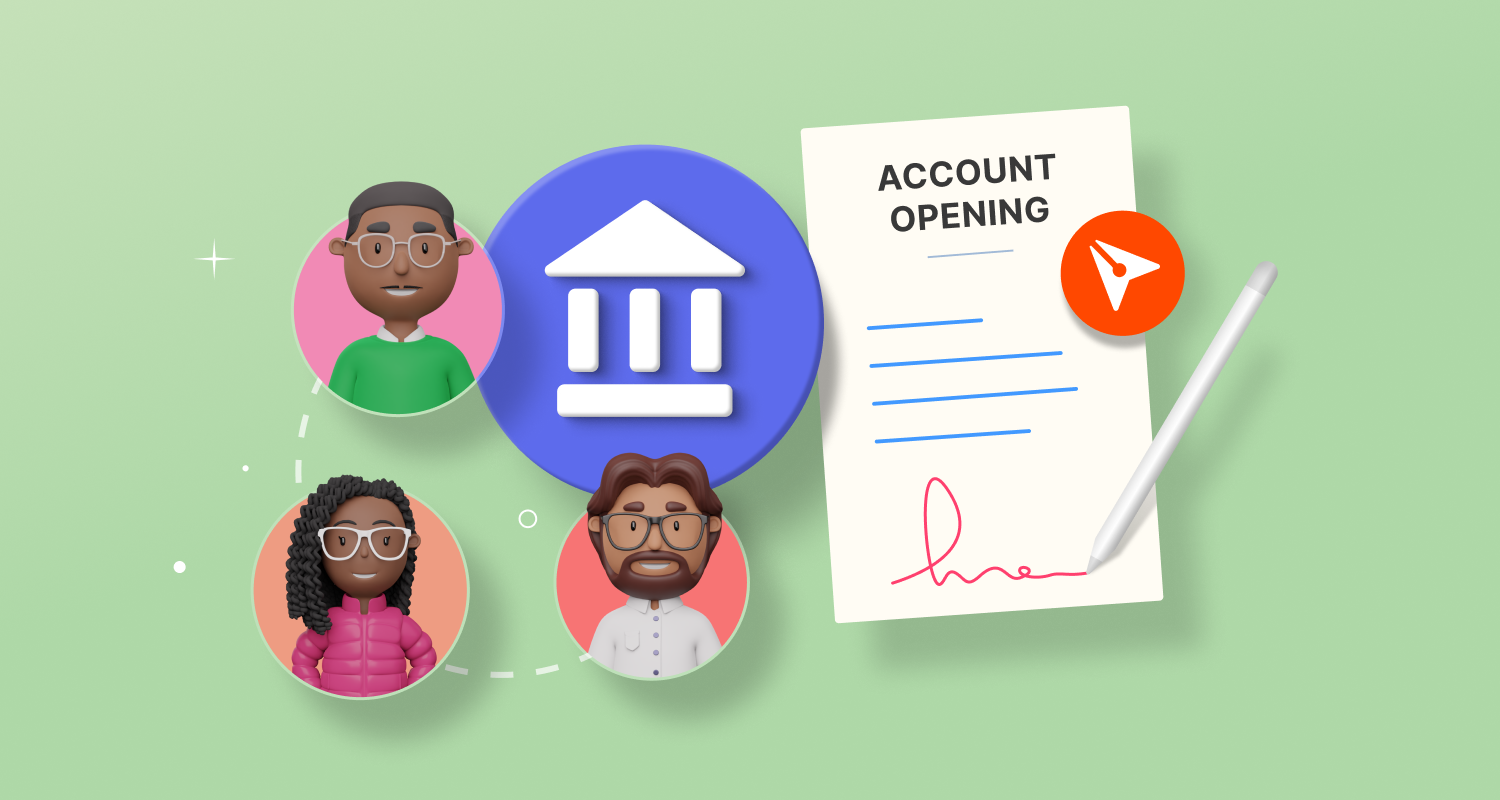
The BoldSign mobile app is now available. Visitthis link for more details and give it a try!
The BoldSign mobile app is now available. Visitthis link for more details and give it a try!
Explore the BoldSign features that make eSigning easier.
Businesses of all sizes rely on written contracts. The average large company juggles tens of thousands of contracts. Managing these by hand is a recipe for delays and mistakes.
The solution is automation. This article explores how to use BoldSign’s API in a C# application to automate the process of generating, sending, and signing employment contracts. BoldSign’s API provides a robust platform for managing electronic signatures and document workflows. The API allows developers to create documents, send them out for signature, and track their status programmatically.
Now our environment is ready.
Pick a PDF document of a contract. It should include standard legal clauses and customizable sections. We can render custom fields on top of the document so the user can read or write the necessary information and then sign the contract.
For simplifying purposes, I divided the code into five sections:
For this step, you only require the previously generated key and a .pdf file containing the body of the document you are sending for review and signature.
I set the key in the code for demo purposes, but I recommend you use a key/vault or an encrypted settings mechanism, so you don’t have to commit the key to your repository.
For this demo, I will use a simple PDF with one space (placeholder) where the signer can enter their electronic signature and a second empty space, which we will be using to populate the signer’s printed name.
A document in BoldSign contains one or more files. You can request a signature for each.
using BoldSign.Api;
using BoldSign.Model;
var apiClient = new ApiClient("https://api.boldsign.com", "[Your API Key]");
var documentClient = new DocumentClient(apiClient);
import requests
import json
url = "https://api.boldsign.com/v1/document/send"
headers = {
'accept': 'application/json',
'X-API-KEY': '[Your API Key]'
}
const axios = require('axios');
const fs = require('fs');
const apiClient = axios.create({
baseURL: 'https://api.boldsign.com',
headers: { 'X-API-KEY': '[Your API Key]' }
});
In this step, we aim to get all the information we need to send our document. We only require the signer’s name and email address for this example.
BoldSign will email the address specified with a link to sign the document securely online. Using the name, we will prepopulate part of the document the signer can’t change.
var signer = new Signer
{
Name = "John Smith",
EmailAddress = "john.smith@gmail.com"
};
signer = {
"name": "John Smith",
'emailAddress': 'john.smith@gmail.com'
}
const signer = {
name: 'John Smith',
emailAddress: 'john.smith@gmail.com'
};
This data is often retrieved from an already persistent data source. In this case, we are just assuming we have the record in memory.
This step is the heart of the operation. It’s where you define the interactive elements of your contract. You’ll determine where they go on the document, whether they’re required, and how they fit into the signing flow.
Once you have decided on your fields, add them to a collection. You’ll need this for the next step: getting that document sent.
Beyond signatures and names, BoldSign offers checkboxes, text boxes, and dates.
var signature = new FormField(
id: "sign",
isRequired: true,
type: FieldType.Signature,
pageNumber: 1,
bounds: new Rectangle(x: 500, y: 740, width: 200, height: 50));
var printedNameField = new FormField(
id: "printedName",
value: signer.Name,
isRequired: true,
type: FieldType.Label,
pageNumber: 1,
bounds: new Rectangle(x: 500, y: 810, width: 200, height: 50));
var formFieldCollections = new List<FormField>()
{
signature,
printedNameField
};
signature = {
"id": "sign",
"name": "string",
"fieldType": "Signature",
"pageNumber": 1,
"bounds": {
"x": 500,
"y": 740,
"width": 200,
"height": 50
},
"isRequired": True
}
printed_name_field = {
"id": "printedName",
"name": "string",
'value': signer['name'],
"fieldType": "Label",
"pageNumber": 1,
"bounds": {
"x": 500,
"y": 810,
"width": 200,
"height": 50
},
"isRequired": True
}
form_field_collections = [signature, printed_name_field]
const signature = {
id: 'sign',
isRequired: true,
fieldType: 'Signature',
pageNumber: 1,
bounds: { x: 500, y: 740, width: 200, height: 50 }
};
const printedNameField = {
id: 'printedName',
value: signer.name,
isRequired: true,
fieldType: 'Label',
pageNumber: 1,
bounds: { x: 500, y: 810, width: 200, height: 50 }
};
const formFieldCollections = [signature, printedNameField];
We are almost done at this point, but wait—how does the signer know they need to sign our contract?
The signers don’t need to have a BoldSign account, but if they create one, they will have access to all the documents they signed and can keep track.
var documentSigner = new DocumentSigner(
signerName: signer.Name,
signerType: SignerType.Signer,
signerEmail: signer.EmailAddress,
formFields: formFieldCollections,
locale: Locales.EN);
var documentSigners = new List<DocumentSigner>()
{
documentSigner
};
var documentFilePath = new DocumentFilePath
{
ContentType = "application/pdf",
FilePath = "my-first-contract.pdf",
};
var filesToUpload = new List<IDocumentFile>
{
documentFilePath
};
var sendForSign = new SendForSign()
{
Message = "Please sign this",
Title = "Agreement",
HideDocumentId = false,
Signers = documentSigners,
Files = filesToUpload
};
signer_data = {
"name": signer['name'],
"emailAddress": signer['emailAddress'],
"signerType": "Signer",
"formFields": form_field_collections,
"locale": "EN"
}
payload = {
'DisableExpiryAlert': 'false',
'ReminderSettings.ReminderDays': '3',
'BrandId': '',
'ReminderSettings.ReminderCount': '5',
'EnableReassign': 'true',
'Message': 'Please sign this.',
'Signers': json.dumps(signer_data),
'ExpiryDays': '10',
'EnablePrintAndSign': 'false',
'AutoDetectFields': 'false',
'OnBehalfOf': '',
'EnableSigningOrder': 'false',
'UseTextTags': 'false',
'SendLinkValidTill': '',
'Title': 'Agreement',
'HideDocumentId': 'false',
'EnableEmbeddedSigning': 'false',
'ExpiryDateType': 'Days',
'ReminderSettings.EnableAutoReminder': 'false',
'ExpiryValue': '60',
'DisableEmails': 'false',
'DisableSMS': 'false'
}
files=[
('Files',('file',open('my-first-contract.pdf','rb'),'application/pdf'))
]
const documentSigner = {
name: signer.name,
signerType: 'Signer',
emailAddress: signer.emailAddress,
formFields: formFieldCollections,
locale: 'en'
};
const FormData = require('form-data');
const form = new FormData();
form.append('Signers', JSON.stringify(documentSigner));
form.append('Title', 'Agreement');
form.append('Message', 'Please sign this.');
form.append('HideDocumentId', 'false');
form.append('Files', fs.readFileSync('my-first-contract.pdf'), {
filename: 'my-first-contract.pdf',
contentType: 'application/pdf'
});
var documentCreated = documentClient.SendDocument(sendForSign);
Console.WriteLine($"Document sent. Id: {documentCreated.DocumentId}");
response = requests.request("POST", url, headers=headers, data=payload, files=files)
print(response.text)
apiClient.post('/v1/document/send', form)
.then(response => {
console.log(`Document sent. Id: ${response.data.documentId}`);
})
.catch(error => {
console.error('Error sending document:', error.response.data);
});
Contracts are foundational for securing services, partnerships, and transactions. Better contract management means securing deals faster and maintaining legal standards with less manual labor. Automating this process reduces the workload and minimizes the risk of human errors.
Raul has been developing backend systems for almost 20 years. He is a Software Engineering Manager by day and a writer by night, sharing his experiences in the field. Raul simplifies software engineering and systems design, helping software engineers.
Raul has been developing backend systems for almost 20 years. He is a Software Engineering Manager by day and a writer by night, sharing his experiences in the field. Raul simplifies software engineering and systems design, helping software engineers.
Latest Articles
How BoldSign Helps with Account Openings in Banking
What’s New in BoldSign: Recent Updates and Features May 2024
How to Set Up Automatic Reminders for eSignature Requests with API