In this article, we will create an Excel function to calculate the distance between two addresses using the Google Maps directions API. This will allow you to get the travel time between the two locations. The format of the function will be as follows: =TRAVELTIME(origin, destination, api_key)
, =TRAVELDISTANCE(origin, destination, apikey)
. The origin and destination will be strings, and can be either an exact address or the name of a place. In order to use the function, an API key is required. The “Getting Started” page can help you with this: http://bit.ly/googlemapsgettingstarted. Create a new project and make sure the Directions API is added.
Step 1: Create a new macro file and add VBA-JSON
Because the Google Maps Directions API is a JSON API, we will use VBA-JSON to make it easy to use the results from the web request. You can download the latest version from here: https://github.com/VBA-tools/VBA-JSON/releases. Download and extract the zip file. Then, open your macro file. Open the Visual Basic Editor (Alt + F11).
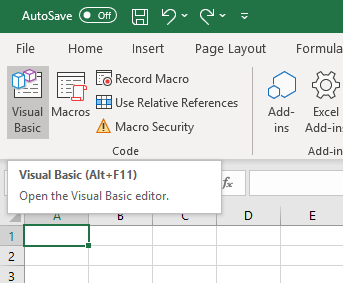
In order to import the VBA-JSON file, go to File > Import File… (Ctrl + M). Select JsonConverter.bas
. A JsonConverter module will appear in the sidebar.

Next, make sure the appropriate references are enabled. Go to Tools > References… In addition to the references already selected, check off “Microsoft Scripting Runtime” (for Dictionary support needed by VBA-JSON) and “Microsoft WinHTTP Services, version 5.1” (to make the HTTP request to the API). If you require support for Excel for Mac, you will need to install VBA-Dictionary from the author of VBA-JSON. More details can be found at the bottom of the project homepage: https://github.com/VBA-tools/VBA-JSON.
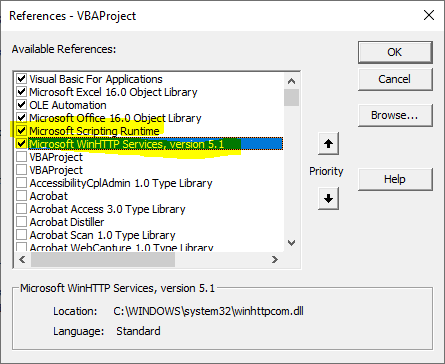
Step 2: Create the functions
With the references configured, we can now write the code for the function. The code is relatively simple. It is simply takes the three parameters and formats them into a web request. The response of the web request is then parsed by VBA-JSON and the relevant variable returned. Note that the request may return multiple routes, but then function simply returns the time of the first route. The default mode is driving, but refer to the Directions API documentation for information on other modes and adjust the strURL variable accordingly.
To insert the code, create a new module with Insert > Module. Then paste the following code:
' Returns the number of seconds it would take to get from one place to another
Function TRAVELTIME(origin, destination, apikey)
Dim strUrl As String
strUrl = "https://maps.googleapis.com/maps/api/directions/json?origin=" & origin & "&destination=" & destination & "&key=" & apikey
Set httpReq = CreateObject("MSXML2.XMLHTTP")
With httpReq
.Open "GET", strUrl, False
.Send
End With
Dim response As String
response = httpReq.ResponseText
Dim parsed As Dictionary
Set parsed = JsonConverter.ParseJson(response)
Dim seconds As Integer
Dim leg As Dictionary
For Each leg In parsed("routes")(1)("legs")
seconds = seconds + leg("duration")("value")
Next leg
TRAVELTIME = seconds
End Function
' Returns the number of seconds it would take to get from one place to another
Function TRAVELDISTANCE(origin, destination, apikey)
Dim strUrl As String
strUrl = "https://maps.googleapis.com/maps/api/directions/json?origin=" & origin & "&destination=" & destination & "&key=" & apikey
Set httpReq = CreateObject("MSXML2.XMLHTTP")
With httpReq
.Open "GET", strUrl, False
.Send
End With
Dim response As String
response = httpReq.ResponseText
Dim parsed As Dictionary
Set parsed = JsonConverter.ParseJson(response)
Dim meters As Integer
Dim leg As Dictionary
For Each leg In parsed("routes")(1)("legs")
meters = meters + leg("distance")("value")
Next leg
TRAVELDISTANCE = meters
End Function
Save the file. You should now be able to use the functions from within Excel. Place your API key in cell A1, then try the following: =TRAVELTIME("24 Sussex Drive Ottawa ON", "Parliament Hill", A1)
. This returns a travel time of about 435 seconds. If you would like this to be displayed in minutes and seconds, try this function: =FLOOR.MATH(A8/60)&" minutes "&MOD(A8, 60)&" seconds"
where A8 is the cell with the travel time in seconds. This prints a helpful “7 minutes 15 seconds” for the 24 Sussex example. We can also find the distance. Try the following: =TRAVELDISTANCE("24 Sussex Drive Ottawa ON", "Parliament Hill", A1)
. It returns a distance of 2667 meters. Convert to kilometers with this: =ROUND(A9/1000, 1)&" km"
.
Note: The Google Maps Directions API always returns distances in meters. Convert to KM or Miles as you wish. This can be done in Excel or by modifying the functions in VBA.
That’s it! You should now have a working travel time function. All you need now is a list of addresses to use it with. If you would like to pull a list from the web or local JSON file, check out Import JSON Data in Excel 2016 or 2019 or Office 365 using a Get & Transform Query.